Python for nodejs developers
Table of contents
🢒Click to expand!
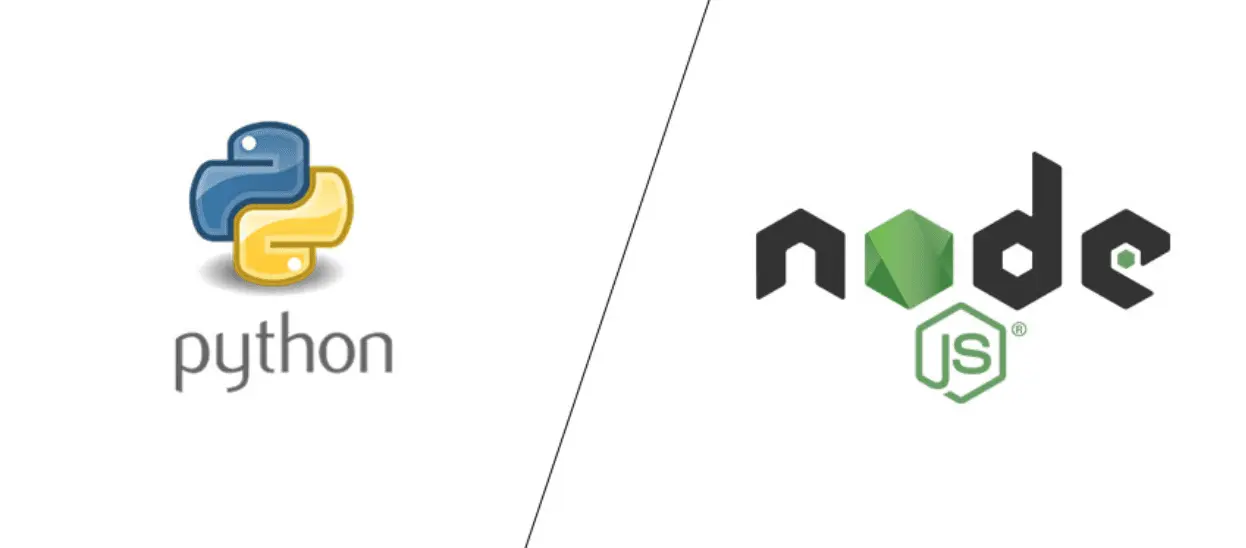
Navigating between programming languages can be a daunting task for developers, especially when transitioning between Python and Node.js. Whether you're considering a switch or simply need to work in both environments, understanding the equivalents and differences between these two powerful languages is crucial for efficient and effective development. This cheatsheet provides a quick reference for developers familiar with either Python or Node.js, aiming to bridge the gap between these two popular languages. This covers the basics
Language Basics
Python | Node.js | Description |
---|---|---|
print("Hello, World!") |
console.log("Hello, World!") |
Output to console |
# This is a comment |
// This is a comment |
Single-line comment |
""" Multi-line string """ |
\` \` \` Multi-line string \` \` \` |
Multi-line string literal |
x = 5 |
let x = 5; |
Variable declaration and assignment |
if condition: |
if (condition) { ... } |
Conditional statement |
for item in iterable: |
for (let item of iterable) { ... } |
Loop over iterable |
def function(): |
function functionName() { ... } |
Function definition |
import module |
const module = require('module'); |
Importing modules |
class MyClass: |
class MyClass { ... } |
Class definition |
Data Types
Python | Node.js | Description |
---|---|---|
int , float , str |
Number , String |
Primitive data types |
list , tuple , dict |
Array , Object |
Data structures |
True , False |
true , false |
Boolean literals |
None |
null , undefined |
Null and undefined values |
Control Flow
Python | Node.js | Description |
---|---|---|
if , elif , else |
if , else if , else |
Conditional statements |
for item in iterable: |
for (let item of iterable) { ... } |
Iterate over arrays, strings, etc. |
while condition: |
while (condition) { ... } |
Loop while condition is true |
try , except , finally |
try , catch , finally |
Exception handling |
Functions
Python | Node.js | Description |
---|---|---|
def function(): |
function functionName() { ... } |
Function definition |
return value |
return value; |
Return value from function |
lambda arguments: expression |
(arguments) => expression |
Anonymous functions (lambda) |
*args , **kwargs |
...args |
Variable-length arguments |
default arguments |
default arguments |
Default parameter values |
global variable |
global variable |
Accessing global variables |
decorators |
decorators |
Function decorators |
Usage
Feel free to refer to this cheatsheet whenever you need to quickly find equivalents between Python and Node.js syntax and concepts.
Contributing
If you find any errors or want to add more content to this cheatsheet, please feel free to fork this repository and submit a pull request. Your contributions are greatly appreciated!